Great resource for formatting WordPress dates and times using the Voxel date format modifier. My goto shortcut.
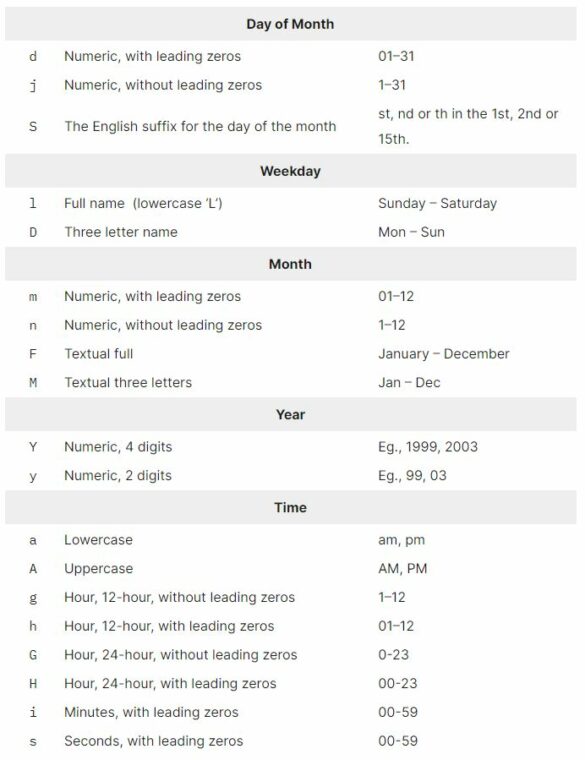
Great resource for formatting WordPress dates and times using the Voxel date format modifier. My goto shortcut.
A great tool to get free open-source SVG icon sets and maintain consistency. Easy to use. Simply bulk-select your desired icons and get a compressed file with all individual SVG icons.
Blazing fast temporary WordPress installations. Great for testing plugin compatibility, and testing site modifications. I use this every day. Could not work without it.
Sometimes you need custom CSS. This CSS reference table demonstrates the different selectors and will help you style your content.
There are no results matching your search.
ResetThe best and most accessible way to achieve this is by using a pseudo element on the link and expanding the clickable area over the entire card.
You need to apply two classes: clickable-card: applied to the card container and clickable-link: applied to the heading that contains the link.
Then copy the CSS from here.
Voxel Elements is faster, while the official Elementor plugin has better compatibility with 3rd party addons.
Voxel Elements is a custom version of the free Elementor page builder plugin, optimized for better performance.
These performance benefits are gained by making changes to the page rendering algorithm, speeding up page loading.
VXE does not make changes to the assets loaded or DOM output.
The Voxel WordPress Theme is an all-in-one framework used for dynamic content, conditional logic, content restrictions, membership, booking, directory and multi-vendor split payments.
See a detailed breakdown here.
Yes. Any custom post types, Elementor data, or any other functionality provided by another theme that follows WordPress standards will keep working when switching to Voxel.
There are no results matching your search.
ResetThere are no results matching your search.
ResetCoffee is my fuel
All templates and guides are available for FREE.
If this saved you time, please support my work by checking out the recommended tools or buying me a coffee.